Dual-wielding is the concept of holding a weapon in each hand instead of a weapon and a shield, or a two-handed weapon. It’s a fun way to give your characters more options for customization. The problem with the way RPG Maker MV handles dual wielding is that there’s no visual feedback from it. Your attack animation still only hits one time, no matter how many weapons you hold.
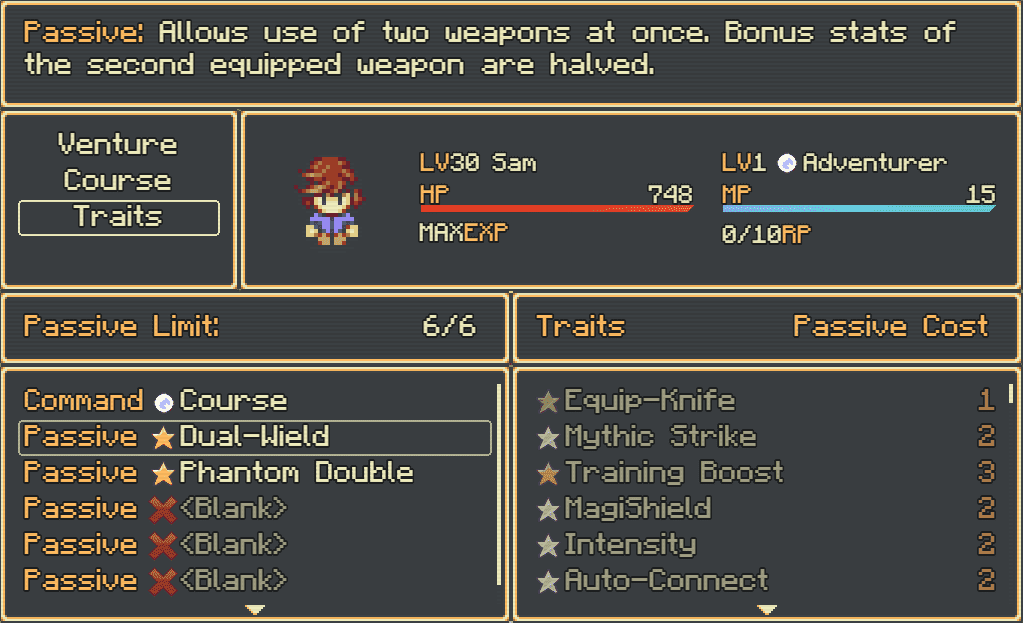
Plugin woes
I’ve been using a plugin to fix this issue. But it’s been giving me grief, randomly changing my character’s stats between each weapon swing and leaving them permanently altered. The plugin’s support was shaky anyway, so rather than spend time troubleshooting, I looked for an alternative.
A practical solution
RPG Maker forums user Arkzein shared a code snippet using Yanfly’s action sequences to simulate a proper dual-wielding effect.
It does what it should quite elegantly, allowing the user to swing each weapon individually and letting those hits have different strengths depending on the weapon’s strength. I added a few extra features to it, including
- a check to see if the weapon fires long-distance, or if the player should approach their target before the attack begins.
- a check to see if the user is carrying two weapons (so it acts like a regular one-hit attack for users with one weapon).
- a check to see if the 2nd weapon has a different animation than the first (otherwise it just repeats the first animation).
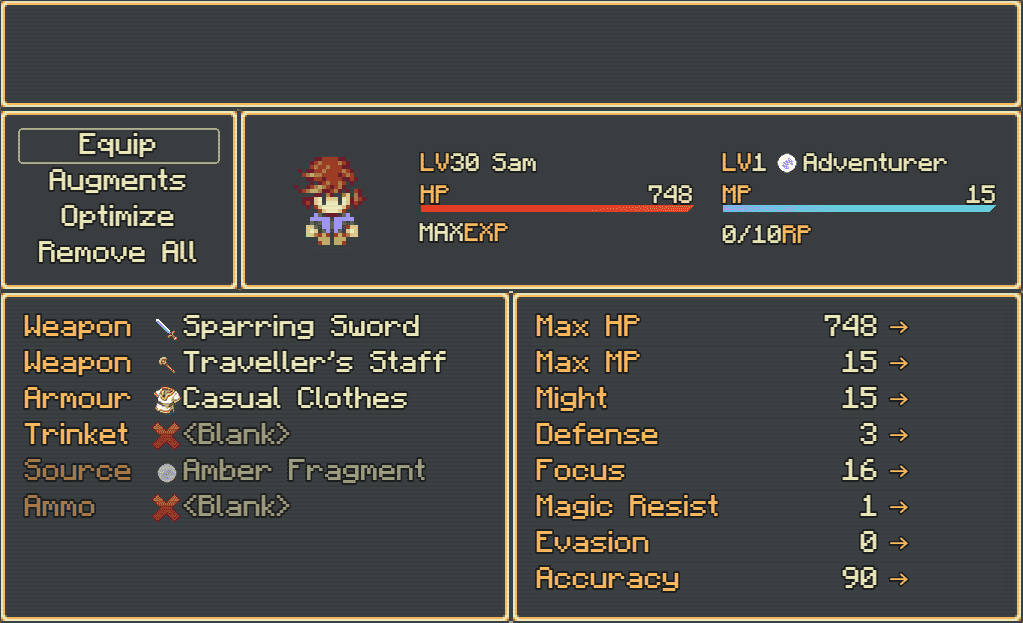
Sample code
Here’s the snippet in full:
// M.o.v.e. forward a little i.f. firing a missile if user.attackMotion() == 'missile' move user: forward, 48, 10 wait for move else // M.o.v.e. to the target move user: target, front base, 10 wait for move end // I.f. the user has multiple weapons + dual-wield if user.weapons().length > 1 && user.isStateAffected(129) EVAL: user._weap1 = user.weapons()[0]; EVAL: user._weap2 = user.weapons()[1]; // Unequips second weapon EVAL: user.forceChangeEquip(1, null); // 1st weapon attack MOTION ATTACK: user MOTION WAIT: user action animation wait for animation action effect EVAL: user.forceChangeEquip(0, user._weap2); // 2nd weapon attack MOTION ATTACK: user MOTION WAIT: user // animation of 2nd weapon EVAL: target.startAnimation(user.weapons()[0].animationId); wait for animation action effect // Restores weapons EVAL: user.forceChangeEquip(0, user._weap1); EVAL: user.forceChangeEquip(1, user._weap2); else // Regular 1 weapon attack MOTION ATTACK: user MOTION WAIT: user action animation wait for animation action effect end
The weird dots in the comments are because action sequences don’t have the concept of comments. So if I didn’t put dots in between the letters of “i.f.” it would be interpreted as a programmatic if statement.